Here is the working solution
create file app/code/Aam/Payment/view/frontend/layout/checkout_index_index.xml
and add code below
<?xml version="1.0"?>
<page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" layout="1column" xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd">
<body>
<referenceBlock name="checkout.root">
<arguments>
<argument name="jsLayout" xsi:type="array">
<item name="components" xsi:type="array">
<item name="checkout" xsi:type="array">
<item name="children" xsi:type="array">
<item name="steps" xsi:type="array">
<item name="children" xsi:type="array">
<!-- New Cart Details Step -->
<item name="cart-details-step" xsi:type="array">
<item name="component" xsi:type="string">Aam_Payment/js/view/checkout-cart-step</item>
<item name="sortOrder" xsi:type="string">0</item> <!-- Display before shipping -->
<item name="children" xsi:type="array">
<!-- Add child components if needed -->
</item>
</item>
</item>
</item>
</item>
</item>
</item>
</argument>
</arguments>
</referenceBlock>
</body>
</page>
2nd step create file
app/code/Aam/Payment/view/frontend/web/js/view/checkout-cart-step.js
define(
[
'ko',
'uiComponent',
'Magento_Checkout/js/model/step-navigator',
'Magento_Customer/js/model/customer',
'Magento_Checkout/js/model/quote',
'Magento_Checkout/js/model/cart/cache'
],
function (ko, Component, stepNavigator, customer, quote, cartCache) {
'use strict';
return Component.extend({
defaults: {
template: 'Aam_Payment/checkout-cart-details'
},
isVisible: ko.observable(true), // Control visibility
stepCode: 'cartdetails',
stepTitle: "Cart Details",
initialize: function () {
this._super();
// Register your step
stepNavigator.registerStep(
this.stepCode,
null,
this.stepTitle,
this.isVisible,
_.bind(this.navigate, this),
0 // Sort order to appear before other steps
);
return this;
},
/**
* The navigate() method can be customized for conditions before switching to this step.
*/
navigate: function () {
// Custom navigation logic, if any
},
/**
* Get cart items
* Use quote.getItems() to fetch items in the cart for display
*/
cartItems: ko.observableArray(quote.getItems()),
/**
* Get totals for display in the cart step
*/
cartTotals: ko.computed(function() {
return quote.totals();
}),
navigateToNextStep: function () {
stepNavigator.next();
}
});
}
);
3rd and last step
app/code/Aam/Payment/view/frontend/web/template/checkout-cart-details.html
<!-- Use 'stepCode' as id attribute -->
<li data-bind="fadeVisible: isVisible, attr: { id: stepCode }">
<div class="step-title" data-bind="i18n: stepTitle" data-role="title"></div>
<div id="checkout-step-title" class="step-content cart-details-step-content" data-role="content">
<div class="fieldset">
<h3 data-bind="i18n: 'Cart Summary'"></h3>
<ul class="cart-items-list" data-bind="foreach: cartItems">
<li class="cart-item">
<span data-bind="text: name"></span>
<span data-bind="text: qty"></span>
<span data-bind="text: price"></span>
</li>
</ul>
<div class="cart-totals">
<span data-bind="i18n: 'Subtotal:'"></span>
<span data-bind="text: cartTotals().subtotal"></span>
</div>
<button type="button" class="action-next-step" data-bind="click: navigateToNextStep, i18n: 'Next'">Next</button>
</div>
</div>
</li>
Do remember to change Aam_Payment with your vendor module name.
Result will be
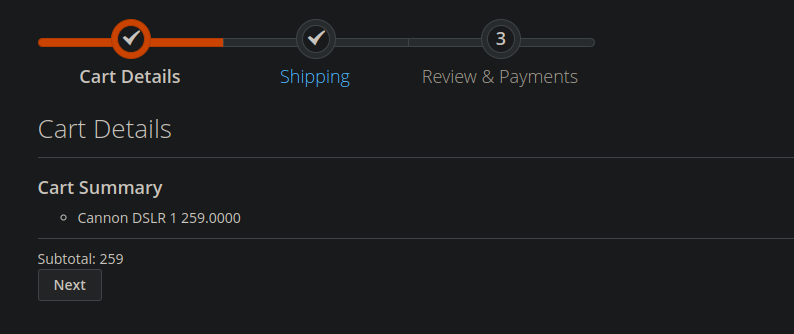