May be there is a better way, for me this worked in (Magento 2.3)
$_product = $this->productloader->create()->load($productId);
$tierPrice = $_product->getTierPrice();
it returns
"tier_price":
[
{
"price_id":"1",
"website_id":"0",
"all_groups":"0",
"cust_group":"0",
"price":85,
"price_qty":"1.0000",
"percentage_value":"15.00",
"website_price":85
},{
"price_id":"2",
"website_id":"0",
"all_groups":"0",
"cust_group":"1",
"price":85,
"price_qty":"1.0000",
"percentage_value":"15.00",
"website_price":85
}
]
Settings in Magento Advanced Pricing:
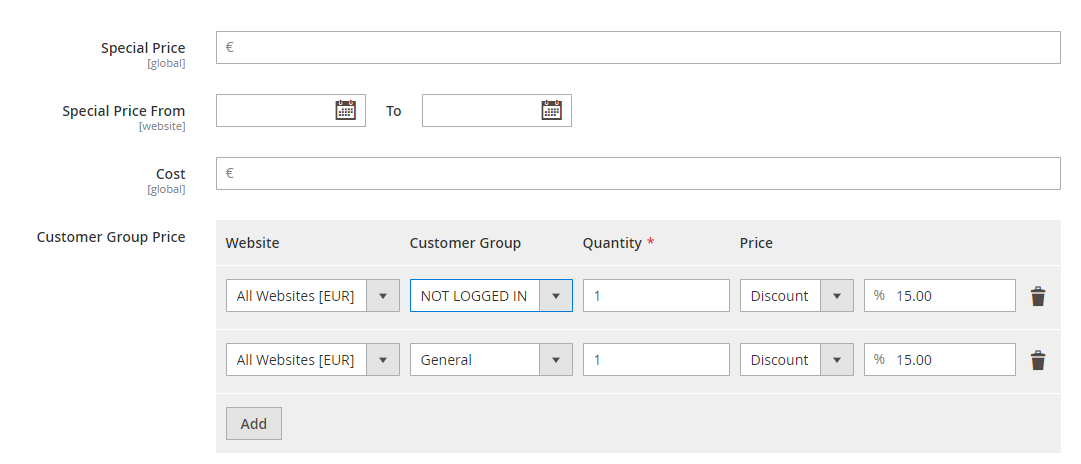